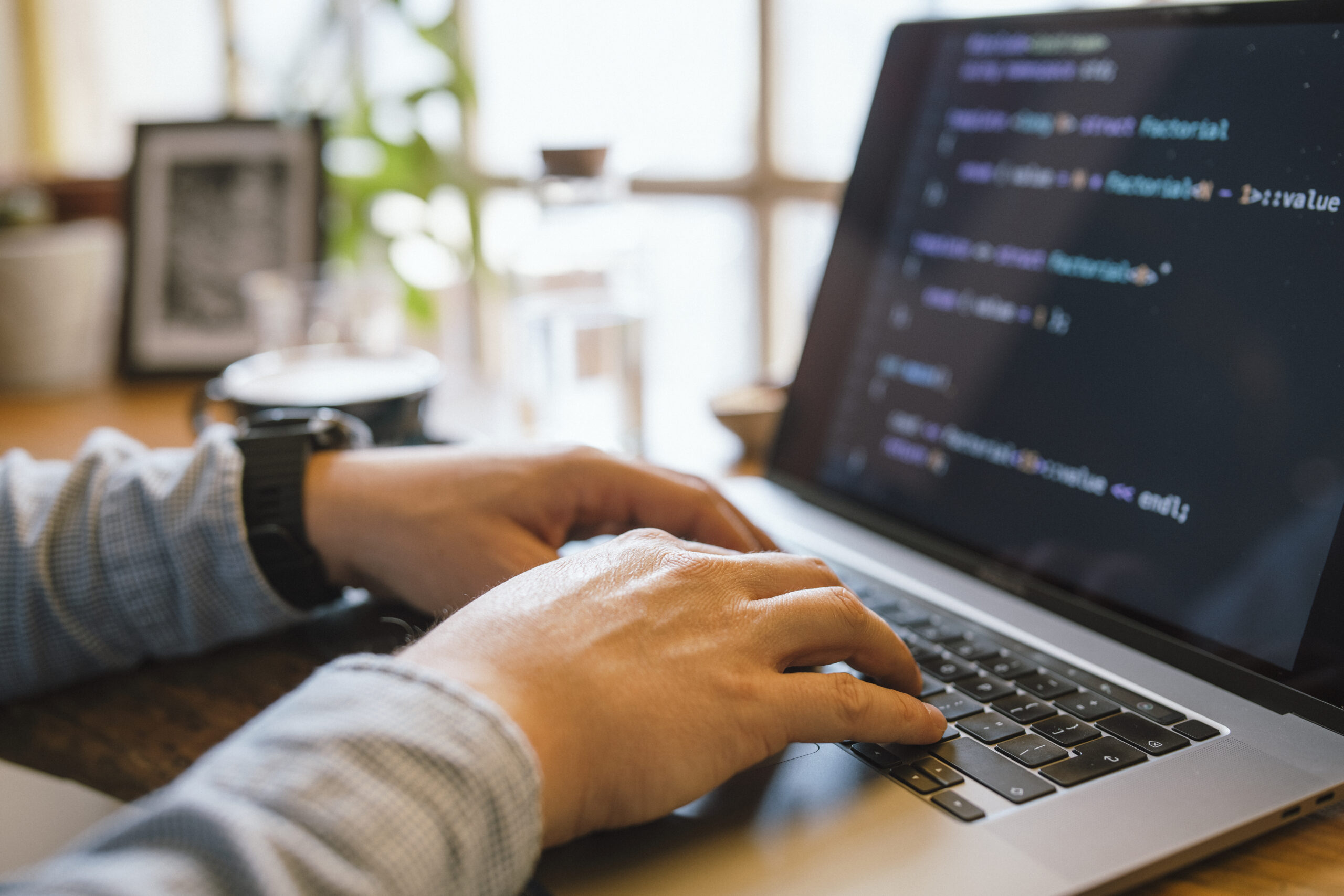
Debugging is Just about the most necessary — however usually neglected — expertise in the developer’s toolkit. It's actually not pretty much correcting damaged code; it’s about being familiar with how and why things go Incorrect, and Studying to Feel methodically to solve difficulties effectively. No matter whether you're a newbie or even a seasoned developer, sharpening your debugging expertise can conserve hours of aggravation and significantly enhance your productivity. Here are several procedures that will help builders amount up their debugging match by me, Gustavo Woltmann.
Grasp Your Resources
One of the fastest strategies developers can elevate their debugging abilities is by mastering the tools they use everyday. When producing code is a single A part of development, recognizing tips on how to interact with it correctly for the duration of execution is equally vital. Modern-day advancement environments come Geared up with effective debugging capabilities — but lots of developers only scratch the area of what these equipment can do.
Acquire, as an example, an Built-in Growth Atmosphere (IDE) like Visible Studio Code, IntelliJ, or Eclipse. These resources assist you to set breakpoints, inspect the worth of variables at runtime, stage as a result of code line by line, and in some cases modify code around the fly. When applied properly, they Permit you to observe just how your code behaves throughout execution, and that is invaluable for tracking down elusive bugs.
Browser developer applications, for example Chrome DevTools, are indispensable for front-conclusion developers. They help you inspect the DOM, keep track of community requests, view true-time overall performance metrics, and debug JavaScript from the browser. Mastering the console, sources, and network tabs can transform annoying UI issues into manageable responsibilities.
For backend or technique-level developers, applications like GDB (GNU Debugger), Valgrind, or LLDB supply deep control about running processes and memory administration. Learning these equipment can have a steeper Understanding curve but pays off when debugging general performance problems, memory leaks, or segmentation faults.
Further than your IDE or debugger, turn into snug with version Manage programs like Git to be aware of code record, find the exact moment bugs had been launched, and isolate problematic alterations.
In the long run, mastering your applications usually means likely beyond default settings and shortcuts — it’s about creating an intimate knowledge of your advancement surroundings to ensure when troubles occur, you’re not dropped at the hours of darkness. The greater you are aware of your applications, the greater time you could expend resolving the particular dilemma as an alternative to fumbling by means of the method.
Reproduce the issue
Just about the most crucial — and often overlooked — ways in productive debugging is reproducing the situation. Ahead of jumping into the code or making guesses, builders need to have to create a consistent environment or state of affairs the place the bug reliably seems. Without having reproducibility, fixing a bug results in being a match of likelihood, often bringing about wasted time and fragile code changes.
The initial step in reproducing a difficulty is gathering just as much context as you possibly can. Talk to issues like: What actions led to The difficulty? Which surroundings was it in — development, staging, or generation? Are there any logs, screenshots, or error messages? The greater detail you have got, the less complicated it gets to be to isolate the precise circumstances less than which the bug happens.
When you’ve gathered sufficient facts, make an effort to recreate the condition in your local ecosystem. This could signify inputting the identical details, simulating equivalent person interactions, or mimicking method states. If The problem seems intermittently, contemplate producing automated exams that replicate the sting cases or condition transitions associated. These tests not merely assistance expose the issue and also prevent regressions Later on.
From time to time, the issue could possibly be ecosystem-particular — it would transpire only on certain working programs, browsers, or less than particular configurations. Making use of instruments like Digital equipment, containerization (e.g., Docker), or cross-browser testing platforms may be instrumental in replicating these kinds of bugs.
Reproducing the situation isn’t simply a step — it’s a state of mind. It calls for endurance, observation, in addition to a methodical approach. But when you can constantly recreate the bug, you are previously midway to repairing it. By using a reproducible circumstance, You should utilize your debugging applications a lot more efficiently, examination likely fixes safely and securely, and talk a lot more Obviously using your crew or end users. It turns an abstract complaint into a concrete obstacle — Which’s wherever builders thrive.
Go through and Realize the Error Messages
Mistake messages will often be the most beneficial clues a developer has when a little something goes Completely wrong. In lieu of observing them as aggravating interruptions, developers should master to take care of error messages as direct communications within the process. They typically let you know precisely what transpired, wherever it took place, and at times even why it happened — if you know the way to interpret them.
Start off by studying the information thoroughly and in full. Quite a few developers, specially when underneath time stress, look at the primary line and instantly get started generating assumptions. But deeper from the error stack or logs may perhaps lie the correct root cause. Don’t just duplicate and paste mistake messages into serps — go through and understand them 1st.
Break the mistake down into parts. Could it be a syntax mistake, a runtime exception, or even a logic mistake? Does it place to a particular file and line range? What module or perform activated it? These concerns can tutorial your investigation and level you towards the accountable code.
It’s also handy to know the terminology with the programming language or framework you’re using. Error messages in languages like Python, JavaScript, or Java generally adhere to predictable designs, and learning to recognize these can considerably quicken your debugging course of action.
Some mistakes are imprecise or generic, As well as in Individuals scenarios, it’s essential to examine the context where the error occurred. Check out similar log entries, input values, and recent improvements within the codebase.
Don’t forget about compiler or linter warnings possibly. These often precede greater troubles and supply hints about potential bugs.
In the end, error messages will not be your enemies—they’re your guides. Learning to interpret them appropriately turns chaos into clarity, supporting you pinpoint difficulties a lot quicker, decrease debugging time, and become a a lot more productive and self-confident developer.
Use Logging Correctly
Logging is One of the more potent resources within a developer’s debugging toolkit. When utilised proficiently, it offers authentic-time insights into how an software behaves, serving to you have an understanding of what’s going on underneath the hood without having to pause execution or move in the code line by line.
A very good logging system starts off with knowing what to log and at what amount. Popular logging concentrations include DEBUG, INFO, Alert, Mistake, and Deadly. Use DEBUG for in depth diagnostic details in the course of advancement, Information for general events (like successful get started-ups), Alert for likely concerns that don’t break the applying, Mistake for precise challenges, and Deadly when the procedure can’t continue on.
Keep away from flooding your logs with extreme or irrelevant data. Far too much logging can obscure significant messages and slow down your system. Center on essential occasions, point out alterations, input/output values, and important determination points in the code.
Format your log messages Evidently and continually. Contain context, such as timestamps, ask for IDs, and function names, so it’s simpler to trace issues in dispersed methods or multi-threaded environments. Structured logging (e.g., JSON logs) will make it even simpler to parse and filter logs programmatically.
All through debugging, logs Allow you to keep track of how variables evolve, what situations are achieved, and what branches of logic are executed—all with no halting This system. They’re Specifically valuable in creation environments where stepping by way of code isn’t possible.
Moreover, use logging frameworks and tools (like Log4j, Winston, or Python’s logging module) that assist log rotation, filtering, and integration with checking dashboards.
In the end, clever logging is about balance and clarity. By using a perfectly-believed-out logging tactic, you are able to lessen the time it takes to spot difficulties, gain deeper visibility into your apps, and Increase the General maintainability and dependability within your code.
Believe Just like a Detective
Debugging is not simply a technological task—it's a kind of investigation. To proficiently identify and resolve bugs, builders ought to solution the process like a detective fixing a thriller. This way of thinking allows break down sophisticated difficulties into workable parts and adhere to clues logically to uncover the root result in.
Start off by collecting proof. Think about the indications of the issue: mistake messages, incorrect output, or effectiveness challenges. Identical to a detective surveys against the law scene, collect as much relevant info as you are able to without having jumping to conclusions. Use logs, check circumstances, and user reviews to piece with each other a clear picture of what’s going on.
Next, variety hypotheses. Talk to you: What can be producing this habits? Have any alterations not long ago been manufactured on the codebase? Has this concern occurred prior to under identical situation? The purpose is usually to narrow down possibilities and detect probable culprits.
Then, examination your theories systematically. Make an effort to recreate the issue in a managed setting. In the event you suspect a selected purpose or element, isolate it and validate if The problem persists. Like a detective conducting interviews, talk to your code inquiries and Allow the results direct you closer to the reality.
Pay out shut consideration to little facts. Bugs usually disguise while in the least predicted locations—similar to a missing semicolon, an off-by-just one error, or maybe a race problem. Be complete and individual, resisting the urge to patch The difficulty without having absolutely comprehension it. Temporary fixes could disguise the true challenge, only for it to resurface later on.
Lastly, preserve notes on Anything you attempted and figured out. Just as detectives log their investigations, documenting your debugging approach can save time for potential difficulties and assist Some others understand your reasoning.
By contemplating similar to a detective, builders can sharpen their analytical expertise, tactic problems methodically, and grow to be simpler at uncovering concealed issues in sophisticated devices.
Publish Checks
Writing exams is one of the best tips on how to improve your debugging expertise and Total progress performance. Tests not only aid catch bugs early but in addition function a security Internet that provides you self esteem when earning changes for your codebase. A nicely-tested application is easier to debug because it enables you to pinpoint specifically the place and when a difficulty happens.
Begin with unit exams, which give attention to personal functions or modules. These little, isolated tests can rapidly reveal whether or not a specific bit of logic is Doing the job as envisioned. Every time a take a look at fails, you quickly know in which to search, substantially decreasing the time used debugging. Device assessments are Specifically helpful for catching regression bugs—problems that reappear after Beforehand currently being mounted.
Subsequent, combine integration assessments and stop-to-finish checks into your workflow. These enable be certain that numerous parts of your software perform with each other effortlessly. They’re notably beneficial for catching bugs that occur in advanced programs with numerous factors or companies interacting. If some thing breaks, your checks can let you know which part of the pipeline unsuccessful and under what ailments.
Creating checks also forces you to Imagine critically about your code. To check a characteristic properly, you require to know its inputs, envisioned outputs, and edge circumstances. This volume of knowing The natural way qualified prospects to raised code construction and less bugs.
When debugging an issue, composing a failing exam that reproduces the bug could be a robust first step. After the take a look at fails regularly, it is possible to focus on repairing the bug and enjoy your test pass when The problem is fixed. This method makes sure that exactly the same bug doesn’t return Sooner or later.
Briefly, writing exams turns debugging from a discouraging guessing game into a structured and predictable approach—serving to you capture much more bugs, more quickly plus much more reliably.
Take Breaks
When debugging a tricky concern, it’s effortless to be immersed in the condition—staring at your display for hrs, striving Option just after Answer. But Just about the most underrated debugging equipment is actually stepping absent. Getting breaks can help you reset your intellect, cut down aggravation, and often see the issue from a new perspective.
When you're as well close to the code for as well lengthy, cognitive fatigue sets in. You could start out overlooking evident glitches or misreading code that you just wrote just hrs previously. On this state, your brain results in being less efficient at problem-resolving. A brief stroll, a coffee break, or even switching to another endeavor for ten–quarter-hour can refresh your emphasis. Several developers report finding the foundation of a dilemma once they've taken time for you to disconnect, letting their subconscious work in the history.
Breaks also enable avert burnout, Specifically throughout longer debugging classes. Sitting in front of a monitor, mentally caught, is not only unproductive but will also draining. Stepping absent enables you to return with renewed Electrical power and also a clearer frame of mind. You may instantly observe a missing semicolon, a logic flaw, or maybe a misplaced variable that eluded you just before.
If you’re caught, a great general guideline is always to established a timer—debug actively for 45–sixty minutes, then take a five–10 moment break. Use that time to maneuver close to, extend, or do one thing unrelated to code. It may well come to feel counterintuitive, especially beneath limited deadlines, nonetheless it actually contributes to more quickly and more effective debugging Over time.
In short, having breaks just isn't an indication of weakness—it’s a smart approach. It presents your brain House to breathe, enhances your standpoint, and aids you steer clear of the tunnel vision That usually blocks your development. Debugging is actually a psychological puzzle, and relaxation is part of fixing it.
Master From Every single Bug
Each bug you come upon is more than just a temporary setback—It truly is a possibility to grow like a developer. Irrespective of whether it’s a syntax error, a logic flaw, or even a deep architectural situation, every one can teach you one thing worthwhile for those who make an effort to reflect and examine what went Erroneous.
Get started by inquiring yourself a couple of crucial inquiries as soon as the bug is fixed: What caused it? Why did it go unnoticed? Could it happen to be caught earlier here with far better techniques like device screening, code opinions, or logging? The responses generally expose blind places in the workflow or being familiar with and help you build stronger coding habits moving ahead.
Documenting bugs will also be a wonderful pattern. Continue to keep a developer journal or retain a log in which you Observe down bugs you’ve encountered, the way you solved them, and That which you uncovered. After a while, you’ll start to see patterns—recurring issues or common issues—you could proactively stay clear of.
In staff environments, sharing Whatever you've realized from a bug with your friends might be Specially effective. Regardless of whether it’s through a Slack information, a brief publish-up, or a quick knowledge-sharing session, encouraging Some others stay away from the exact same difficulty boosts staff effectiveness and cultivates a stronger Discovering lifestyle.
Much more importantly, viewing bugs as classes shifts your frame of mind from aggravation to curiosity. Instead of dreading bugs, you’ll start out appreciating them as crucial parts of your growth journey. In the end, a lot of the ideal builders will not be those who publish perfect code, but people that constantly study from their blunders.
Eventually, Each and every bug you take care of adds a different layer to your ability established. So subsequent time you squash a bug, have a instant to reflect—you’ll appear absent a smarter, a lot more able developer because of it.
Conclusion
Increasing your debugging skills normally takes time, observe, and patience — even so the payoff is large. It tends to make you a more successful, confident, and capable developer. The following time you happen to be knee-deep inside of a mysterious bug, keep in mind: debugging isn’t a chore — it’s a chance to be improved at Everything you do.