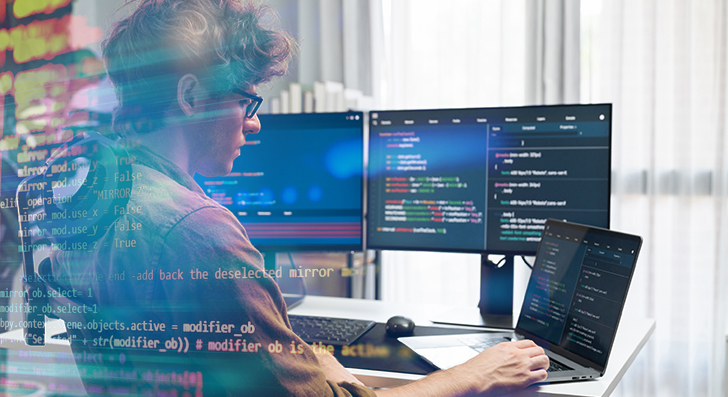
Scalability signifies your software can cope with expansion—a lot more customers, extra facts, plus much more targeted traffic—without having breaking. As a developer, making with scalability in mind will save time and pressure later. Below’s a clear and realistic guidebook that will help you get started by Gustavo Woltmann.
Layout for Scalability from the Start
Scalability isn't really some thing you bolt on afterwards—it should be aspect of one's system from the beginning. Quite a few applications fall short when they increase quickly for the reason that the initial structure can’t manage the additional load. As being a developer, you'll want to think early about how your procedure will behave under pressure.
Start off by designing your architecture for being adaptable. Steer clear of monolithic codebases the place everything is tightly connected. As a substitute, use modular design or microservices. These patterns split your application into smaller, independent areas. Each individual module or services can scale on its own devoid of influencing the whole program.
Also, take into consideration your database from working day a person. Will it need to deal with 1,000,000 end users or perhaps a hundred? Select the suitable style—relational or NoSQL—according to how your info will increase. System for sharding, indexing, and backups early, Even when you don’t have to have them yet.
An additional crucial position is to stop hardcoding assumptions. Don’t produce code that only is effective less than present-day conditions. Consider what would occur In case your user base doubled tomorrow. Would your application crash? Would the databases decelerate?
Use style and design designs that assist scaling, like concept queues or function-driven methods. These assist your app handle more requests without having overloaded.
After you Establish with scalability in your mind, you are not just planning for success—you're reducing upcoming problems. A properly-planned method is less complicated to take care of, adapt, and increase. It’s far better to prepare early than to rebuild afterwards.
Use the best Database
Choosing the ideal database is really a key Component of constructing scalable programs. Not all databases are built the exact same, and using the wrong you can slow you down or simply bring about failures as your app grows.
Get started by comprehension your information. Can it be very structured, like rows in a desk? If Sure, a relational databases like PostgreSQL or MySQL is an effective fit. These are definitely sturdy with relationships, transactions, and consistency. In addition they assist scaling techniques like examine replicas, indexing, and partitioning to handle additional visitors and details.
Should your details is much more adaptable—like consumer activity logs, merchandise catalogs, or files—contemplate a NoSQL possibility like MongoDB, Cassandra, or DynamoDB. NoSQL databases are far better at managing big volumes of unstructured or semi-structured knowledge and will scale horizontally a lot more conveniently.
Also, think about your examine and write designs. Will you be doing a great deal of reads with much less writes? Use caching and skim replicas. Have you been dealing with a major create load? Investigate databases which can deal with large produce throughput, or simply occasion-dependent details storage systems like Apache Kafka (for short-term info streams).
It’s also clever to Imagine in advance. You may not require Superior scaling characteristics now, but deciding on a databases that supports them usually means you received’t need to switch later.
Use indexing to speed up queries. Steer clear of needless joins. Normalize or denormalize your knowledge dependant upon your entry designs. And generally watch databases effectiveness when you improve.
Briefly, the appropriate databases will depend on your application’s framework, pace wants, And the way you anticipate it to develop. Take time to select sensibly—it’ll help save many difficulties later on.
Optimize Code and Queries
Quickly code is key to scalability. As your application grows, each individual smaller hold off adds up. Poorly penned code or unoptimized queries can decelerate functionality and overload your program. That’s why it’s crucial to build economical logic from the beginning.
Commence by writing clean up, uncomplicated code. Keep away from repeating logic and remove anything unwanted. Don’t select the most complex Alternative if an easy 1 is effective. Maintain your functions small, targeted, and easy to check. Use profiling tools to uncover bottlenecks—spots exactly where your code usually takes way too long to operate or utilizes far too much memory.
Future, have a look at your databases queries. These typically slow factors down more than the code by itself. Make sure Each individual query only asks for the information you actually need to have. Avoid Decide on *, which fetches everything, and alternatively select distinct fields. Use indexes to hurry up lookups. And steer clear of executing too many joins, Specially throughout big tables.
In case you notice the identical facts being requested time and again, use caching. Store the outcomes briefly applying tools like Redis or Memcached and that means you don’t really have to repeat expensive operations.
Also, batch your database functions any time you can. Instead of updating a row one by one, update them in groups. This cuts down on overhead and can make your application extra efficient.
Remember to check with massive datasets. Code and queries that get the job done great with 100 records may crash after they have to manage one million.
Briefly, scalable applications are speedy applications. Keep your code tight, your queries lean, and use caching when needed. These actions aid your application remain easy and responsive, even as the load raises.
Leverage Load Balancing and Caching
As your app grows, it has to handle much more customers and even more site visitors. If almost everything goes by way of one particular server, it is going to speedily become a bottleneck. That’s in which load balancing and caching are available in. These two tools help keep the application rapidly, steady, and scalable.
Load balancing spreads incoming site visitors across multiple servers. In lieu of a person server executing every one of the operate, the load balancer routes consumers to various servers based on availability. This suggests no solitary server gets overloaded. If one server goes down, the load balancer website can mail visitors to the Other individuals. Tools like Nginx, HAProxy, or cloud-centered remedies from AWS and Google Cloud make this simple to set up.
Caching is about storing details briefly so it can be reused immediately. When end users request a similar data once more—like a product site or even a profile—you don’t need to fetch it with the database when. It is possible to serve it through the cache.
There are two prevalent varieties of caching:
one. Server-side caching (like Redis or Memcached) suppliers info in memory for speedy entry.
two. Consumer-facet caching (like browser caching or CDN caching) retailers static data files close to the user.
Caching lowers database load, enhances velocity, and tends to make your application much more successful.
Use caching for things which don’t modify normally. And often be certain your cache is up to date when facts does change.
In a nutshell, load balancing and caching are very simple but effective instruments. Together, they help your application tackle a lot more people, stay quickly, and Get well from complications. If you plan to increase, you would like each.
Use Cloud and Container Equipment
To develop scalable purposes, you'll need equipment that allow your application mature effortlessly. That’s in which cloud platforms and containers can be found in. They offer you flexibility, decrease setup time, and make scaling Considerably smoother.
Cloud platforms like Amazon World-wide-web Services (AWS), Google Cloud Platform (GCP), and Microsoft Azure let you rent servers and solutions as you will need them. You don’t really need to obtain components or guess long run potential. When targeted visitors increases, you can add much more sources with just a few clicks or instantly making use of automobile-scaling. When site visitors drops, you'll be able to scale down to save cash.
These platforms also provide solutions like managed databases, storage, load balancing, and security tools. You can focus on building your application in place of taking care of infrastructure.
Containers are One more crucial Instrument. A container packages your application and anything it should run—code, libraries, configurations—into just one device. This makes it easy to maneuver your app in between environments, from your notebook for the cloud, with out surprises. Docker is the most popular Resource for this.
Whenever your app works by using a number of containers, resources like Kubernetes assist you take care of them. Kubernetes handles deployment, scaling, and recovery. If one aspect of the application crashes, it restarts it routinely.
Containers also allow it to be straightforward to independent parts of your application into solutions. You can update or scale sections independently, which can be perfect for efficiency and trustworthiness.
In a nutshell, using cloud and container instruments indicates you may scale quick, deploy quickly, and Recuperate promptly when issues transpire. If you would like your application to grow with no limits, commence applying these resources early. They help save time, reduce chance, and assist you remain centered on setting up, not fixing.
Check All the things
In the event you don’t keep an eye on your software, you received’t know when issues go Mistaken. Checking helps you see how your app is undertaking, location problems early, and make far better selections as your application grows. It’s a vital A part of constructing scalable devices.
Get started by tracking fundamental metrics like CPU usage, memory, disk Room, and reaction time. These inform you how your servers and products and services are doing. Resources like Prometheus, Grafana, Datadog, or New Relic will help you acquire and visualize this knowledge.
Don’t just watch your servers—observe your application much too. Regulate how much time it takes for users to load pages, how often errors happen, and exactly where they happen. Logging equipment like ELK Stack (Elasticsearch, Logstash, Kibana) or Loggly may help you see what’s occurring inside your code.
Create alerts for crucial difficulties. By way of example, Should your response time goes over a limit or a service goes down, you should get notified immediately. This allows you take care of difficulties rapid, typically just before customers even discover.
Checking is likewise valuable once you make modifications. If you deploy a completely new element and see a spike in errors or slowdowns, you could roll it back again just before it causes serious hurt.
As your app grows, targeted visitors and knowledge improve. Without checking, you’ll skip indications of problems until it’s far too late. But with the correct tools in position, you stay on top of things.
In short, checking assists you keep the app trusted and scalable. It’s not nearly recognizing failures—it’s about knowing your procedure and ensuring it really works effectively, even stressed.
Last Feelings
Scalability isn’t just for massive companies. Even modest applications want a solid foundation. By coming up with carefully, optimizing correctly, and utilizing the correct instruments, you are able to Create applications that expand efficiently without breaking under pressure. Start out little, Consider significant, and Develop clever.